Getting Started
This guide walks you through getting started with the TrustCloud API. The TrustCloud API is a RESTful API that enables companies to programmatically interact with their TrustCloud to retrieve data about their governance, risk, and compliance program, and to submit evidence to satisfy evidence requirements associated with controls.
Setup
How do I generate a new API key?
Generating an API Key consists of three steps:- Before You Begin,
- Configure API Key, and
- Complete Setup.
- Navigate to the Account Summary page.
- Click on the Admin menu from the left-side panel.
- Click on API Access option from Admin menu.
Note: The Account Summary page is accessible only to the Compliance Admin role.
- On the API Access page, click on the Begin Setup button
- The Before You Begin page is displayed
Before You Begin
This step states the capabilities of the Trustcloud API and provides documentation to help you complete your API setup.- Click on the “Configure API Key” button.
- The Configure API Key page is displayed.
Configure API Key
This step lets you enter the information related to the API Key.- Enter the API Key Name and set the key expiration period.
- Click on the “Generate Key” button.
- Copy the API key
- Click on the “Complete Setup” button.
Complete Setup
This step lets you set a contact email address to get notifications related to API and related tasks.- Enter the Contact Email to receive notification emails related to the expiration of keys.
- Click on the “Finish” button, and the API key listing page will be displayed. You can change the Contact information on the Contact Info tab.
- You can delete the existing key by clicking on the “Delete” icon next to the key.
What if the API key is already present?
On the API Access page, as an administrator, you can view the list of API keys on the API Keys tab. You can add a new API key by clicking on the “Add API Key” button. You cannot add an API key that is already present in the list. If you try to add any existing API key name, an error message is displayed, as shown in the following screenshot.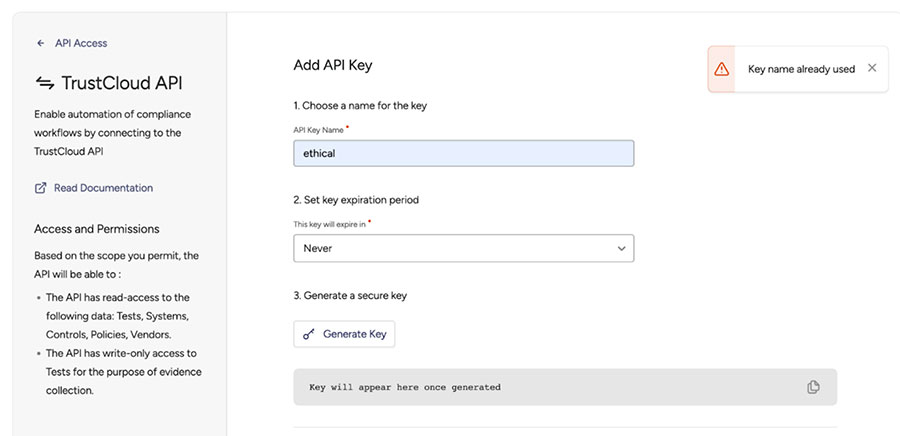
Authenticating Requests
All requests are authenticated with the API key by including it as a Bearer token in the request’s Authorization header.
Authorization: Bearer
API Versioning
The API is versioned via the x-trustcloud-api-version header, which is required.
X-trustcloud-api-version 1
Currently, the TrustCloud API is at version 1.
Writing your first API Request
You can validate your connection to the TrustCloud API by sending a request to retrieve information about your API Key:
const axios = require('axios');
async function getApiKey() {
const apiKey = '';
const apiUrl = 'https://api.trustcloud.ai/apikeys/me';
try {
const response = await axios.get(apiUrl, {
headers: {
'Authorization': `Bearer ${apiKey}`,
'x-trustcloud-api-version': '1'
}
});
console.log(response.data);
} catch (error) {
console.error(error);
}
}
import java.io.IOException;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
public class TrustCloudAPITest {
public static void main(String[] args) throws IOException, InterruptedException {
String apiKey = "";
HttpClient httpClient = HttpClient.newBuilder()
.version(HttpClient.Version.HTTP_1_1)
.build();
HttpRequest request = HttpRequest.newBuilder()
.GET()
.uri(java.net.URI.create("https://api.trustcloud.ai/apikeys/me"))
.setHeader("Authorization", "Bearer " + apiKey)
.setHeader("x-trustcloud-api-version", "1")
.build();
HttpResponse response = httpClient.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println(response.body());
}
}
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Threading.Tasks;
namespace TrustCloudAPIExample
{
class Program
{
static async Task Main(string[] args)
{
var apiKey = "";
var httpClient = new HttpClient();
httpClient.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", apiKey);
httpClient.DefaultRequestHeaders.Add("x-trustcloud-api-version", "1");
var response = await httpClient.GetAsync("https://api.trustcloud.ai/apikeys/me");
var responseBody = await response.Content.ReadAsStringAsync();
Console.WriteLine(responseBody);
}
}
}
import requests
def get_api_key():
api_key = ''
api_url = 'https://api.trustcloud.ai/apikeys/me'
headers = {
'Authorization': f'Bearer {api_key}',
'x-trustcloud-api-version': '1'
}
response = requests.get(api_url, headers=headers)
print(response.json())
package main
import (
"fmt"
"io/ioutil"
"net/http"
)
func getApiKey() {
apiKey := ""
apiUrl := "https://api.trustcloud.ai/apikeys/me"
req, err := http.NewRequest("GET", apiUrl, nil)
if err != nil {
fmt.Println("Error creating HTTP request:", err)
return
}
req.Header.Set("Authorization", fmt.Sprintf("Bearer %s", apiKey))
req.Header.Set("x-trustcloud-api-version", "1")
client := &http.Client{}
resp, err := client.Do(req)
if err != nil {
fmt.Println("Error sending HTTP request:", err)
return
}
defer resp.Body.Close()
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
fmt.Println("Error reading response body:", err)
return
}
fmt.Println(string(body))
}
GET /apikeys/me HTTP/1.1
Host: API.trustcloud.ai
Authorization: [your_api_key_here]
x-trustcloud-api-version: 1
This will return a payload with your API key details:
HTTP/1.1 200 OK
Content-Type: application/json
{
"keyName": "[The name of my API Key]",
"expiresAt": "[Date and time of key expiration"
}
What’s next?
Congratulations, you’ve now connected to TrustCloud API! To continue, review our guides on Security, Retrieving Trust Objects and Submitting Evidence. Or, check out our API Guide.